단위 테스트를 이용하면 디버그 테스트를 통해 소스 생성기 소스코드에 중단점을 지정해 디버깅이 가능합니다.
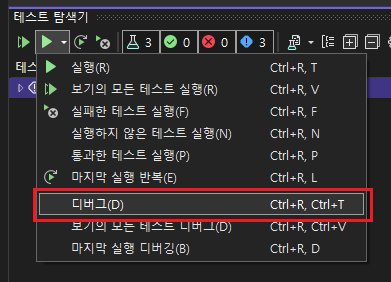
![image]()
이제 TextAttribute
를 통해 ToStringFast()
로 반환될 텍스트를 지정해보려 합니다.
[UsesVerify]
public class EnumGeneratorTests
{
[Fact]
public Task TestAutogenEnum()
{
var source = """
using Autogen.Enum;
[AutogenEnum]
public enum Color
{
Red,
Blue,
}
""";
return TestHelper.Verify(source);
}
[Fact]
public Task TestAutogenEnumWithTextAttribute1()
{
var source = """
using Autogen.Enum;
[AutogenEnum]
public enum Color
{
[Text("빨강")]
Red = 0,
[Text("파랑")]
Blue = 1,
}
""";
return TestHelper.Verify(source);
}
[Fact]
public Task TestAutogenEnumWithTextAttribute2()
{
var source = """
using Autogen.Enum;
[AutogenEnum]
public enum Color
{
[Text("빨강")]
Red = 0,
Blue = 1,
[Text("노랑")]
Yellow = 2,
}
""";
return TestHelper.Verify(source);
}
}
Verify
의 스냅샷으로 테스트를 진행할 수 있으므로 테스트 코드가 한결 깔끔합니다. Verify
에 의해 생성된 파일이 올바름을 확인하면
생성된 *.received.cs
를 *.verified.cs
로 바꿔주는 것으로 검증이 완료됩니다.
| 샘플) EnumGeneratorTests.TestAutogenEnumWithTextAttribute2.01.verified.cs
//HintName: Autogen.Enum.EnumExtensions.g.cs
namespace Autogen.Enum
{
public static partial class EnumExtensions
{
public static string ToStringFast(this Color value) => value switch
{
Color.Red => "빨강",
Color.Blue => nameof(Color.Blue),
Color.Yellow => "노랑",
_ => value.ToString(),
};
}
}
모든 테스트가 통과되었습니다.
![image]()
적절하게 프로젝트 참조를 하고,
| AutogenEnum.Sample.proj
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<OutputType>Exe</OutputType>
<TargetFramework>net6.0</TargetFramework>
<ImplicitUsings>enable</ImplicitUsings>
<Nullable>enable</Nullable>
</PropertyGroup>
<ItemGroup>
<ProjectReference Include="..\..\src\Autogen.Core\Autogen.Core.csproj" OutputItemType="Analyzer" ReferenceOutputAssembly="false" />
<ProjectReference Include="..\..\src\Autogen.Enum\Autogen.Enum.csproj" OutputItemType="Analyzer" ReferenceOutputAssembly="false" />
<ProjectReference Include="..\..\src\Autogen.SourceGeneration\Autogen.SourceGeneration.csproj" OutputItemType="Analyzer" ReferenceOutputAssembly="false" />
</ItemGroup>
</Project>
| Program.cs
using Autogen.Enum;
var w1 = Week.Sunday;
Console.WriteLine(w1.ToStringFast());
var w2 = Week.Monday;
Console.WriteLine(w2.ToStringFast());
[AutogenEnum]
public enum Week
{
[Text("일요일")]
Sunday,
Monday,
}
| 결과
일요일
Monday
그런데 실제 컴파일은 잘되지만 다음처럼 IDE 화면에 컴파일 오류가 메시지가 표시됩니다.
![image]()
제가 Analyzer DLL 참조 방식이라던가 환경을 몰라 아직은 이 문제를 해결하지 못하고 있습니다.
전체 소스